XP Dialog Box
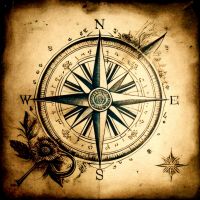
Hello! I'm learning XP. It's fun, and it may turn out to be a critical tool for me. I could not find the answer to a question anywhere, so I thought I'd ask here.
How can one create a custom dialog window from C++? I can input text or use the common dialogs, but I'd like to collect multiple inputs like some of the internal tools. For instance, a few integers, perhaps a color, etc.
Thank you!
Comments
The short version for FastCAD custom dialogs is:
A short example of a command that uses a dialog:
And here's the corresponding dialog resource:
Yay. Thank you. I will try this.
OK, I have been working with this for a bit. I hate to come back to the forums for help with something that should be simple, but I can't find anything on Google to help.
Do you have any documentation on DefDlg?
When I call it (e.g., your line #31), it returns a handle. It returns the handle even when I pass in a garbage dialog name, as though that first parameter isn't being used correctly. The handle that comes back always causes a return of 1 (IDOK) from XPDlog. It never calls the init function (e.g., initDialog from your example). Any documentation for that DefDlg function might help me.
I'll admit, it's been a long time since I worked with Win32 dialog code. Please forgive my silly questions. I really appreciate the help! Thank you!
You need to make sure that your dialog resource is named as a string rather than the default enumeration that the Visual Studio rc file editor generates. The FastCAD engine has a feature that strings specified as smallish numbers (resource IDs) get converted internally in its translation lookup table. Unfortunately, enumerations generated by the VS resource editor fall right into that range.
Short version: delete the #define in resource.h that corresponds to your dialog and it should start working. I tend to be mystified by this regularly, so it's not just you.
Technical note: DefDlg returns the address of a FastCAD dialog record that includes (among other things) the Windows dialog handle. Failure to load the resource isn't well communicated and calling xpdlg with an incompletely initialized dialog record returns immediately with a "I did everything I could so all is well" return value.
Brilliant. Thank you for the tip. That explains everything.